🍋 문제링크
https://www.acmicpc.net/problem/1406
🍎 코드 제출 기록 (메모리 및 시간)
메모리 : 2808 KB
시간 : 40 ms
🍉 Code
#include <iostream>
#include <stack>
int N;
std::stack <char> left;
std::stack <char> right;
std::stack <char> tmp;
void input_faster()
{
std::ios_base::sync_with_stdio(false);
std::cin.tie(0);
std::cout.tie(0);
}
void input()
{
std::string str;
std::cin >> str;
for(int i = 0; i < str.size() ; i++){
left.push(str[i]);
}
std::cin >> N;
}
void solve()
{
char func;
while (N--){
std::cin >> func;
if (func == 'P'){
char x;
std::cin >> x;
left.push(x);
}
else if (func == 'L'){
if (!left.empty()){
right.push(left.top());
left.pop();
}
}
else if (func == 'B'){
if (!left.empty())
left.pop();
}
else if (func == 'D'){
if (!right.empty()){
left.push(right.top());
right.pop();
}
}
}
}
void print_val()
{
while (!left.empty()){
tmp.push(left.top());
left.pop();
}
while (!tmp.empty()){
std::cout << tmp.top();
tmp.pop();
}
while (!right.empty()){
std::cout << right.top();
right.pop();
}
}
int main()
{
input_faster();
input();
solve();
print_val();
return (0);
}
🥝 메모
문자열에서 커서를 이동하고 중간에 문자를 삽입하는 과정을 쉽게 만들기 위해 stack 두개를 사용하였습니다.
편의상 두 스택의 이름을 left, right 라고 하고
이해를 위해 right 스택의 좌우를 반전하여 그리겠습니다!
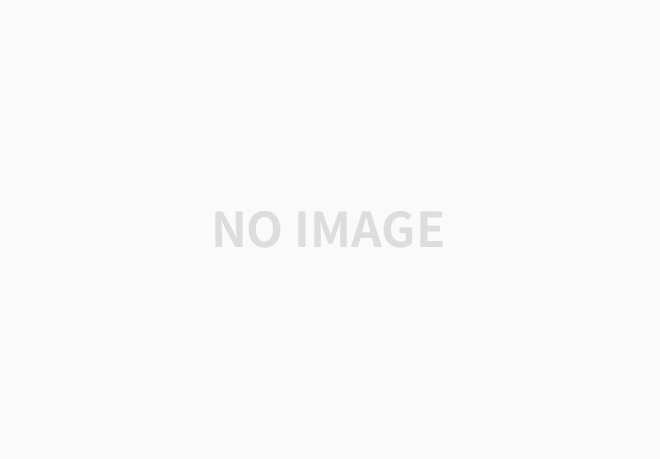
각각의 연산을 그림으로 표현하면 아래와 같고
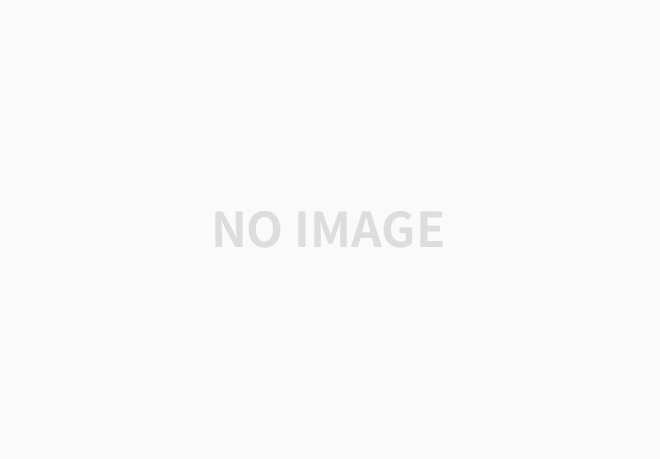
💥 각 연산에서 스택이 비어있는지 확인을 하고! pop!을 하는건 절대 잊으면 안됩니닷! ㅎㅎ
반응형
'➰ 취업준비 > 알고리즘 문제풀이' 카테고리의 다른 글
[C++][백준][1260] DFS와 BFS (DFS/BFS) (0) | 2021.04.12 |
---|---|
[Python][백준][2003] 수들의 합 2 (투 포인터) (0) | 2021.04.08 |
[Python][백준][11057] 오르막 수 (DP) (0) | 2021.04.07 |
[Python][백준][1874] 스택 수열 (STACK) (0) | 2021.04.01 |
[Python][백준][2294] 동전 2 (DP) (0) | 2021.03.29 |